Adding Sendgrid to Remix.js
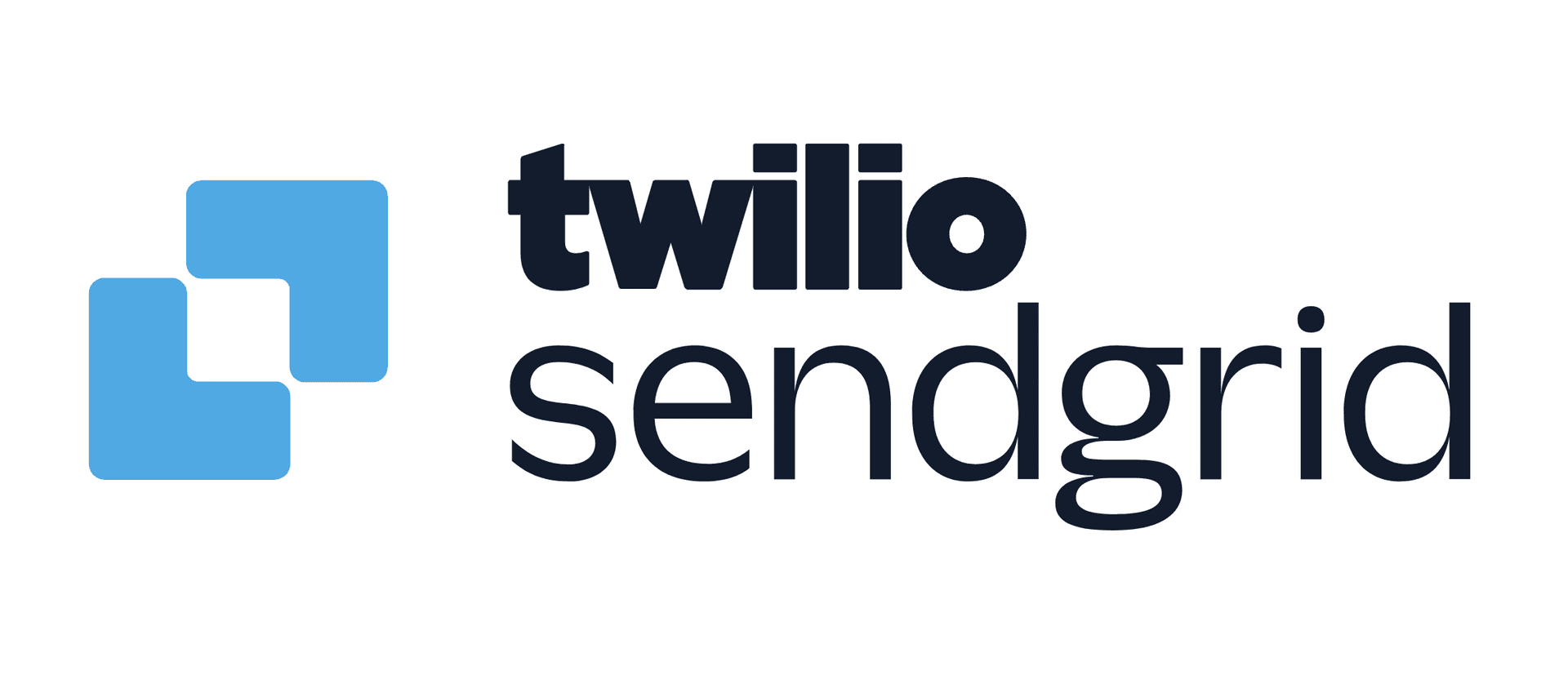
Before we get started with building a contact page using SendGrid within the Remix.js framework, let’s first discuss how incredibly simple it was to convert my static pages from my Next.js portfolio website.
This is a continuation of my experimental series on using Remix.js instead of Next.js. You can read the first blog, Using Google Fonts with Remix.js, TailwindCSS, and Fontsource, where I implemented an efficient way of achieving near font optimization in Remix.js, which is a feature that is lacking compared to Next.js.
After setting up Google Fonts for my style guide using TailwindCSS configuration, I proceeded to configure the rest of my style guide in TailwindCSS.

As I mentioned earlier, converting the static pages was really simple. On my portfolio website, I have three static pages: the homepage, the “about me” page, and the services page. Since I’m using TailwindCSS and all the styles are so-called “inlined,” all I had to do was copy and paste the JSX code from my three files. The only updates I needed to make to convert from Next.js to Remix.js were the imported modules, specifically for the Link and Meta modules. This was a simple swap:
The Next.js imports:

The Remix.js imports:

Once I swapped those imports, the pages loaded up. I must say, working in the local environment for Remix.js is significantly faster. This was my first major realization of a key difference between Remix.js and Next.js.
Now, back to the core of this article. This was another big finding: it was much simpler to code out my contact page. The amount of code I had to write was reduced by hundreds of lines. I could have probably split up my code into different files, but remember, this is just an experimental project, and I’m not aiming for a production-ready website with all the best practices.
In Next.js, to accomplish this, I had to write a ton of code and even create an API route to call the SendGrid API. Let’s just say it was a learning process and not as straightforward as Remix.js.
With Remix.js, the first thing I did was install the SendGrid package into my project.


Next, I created a file under the routes folder called contact.tsx. This is where I set up my contact form. Once again, I just copied and pasted the code from my Next.js contact file. The only updates I had to make were to the imported modules. The key imports to focus on are ActionFunction, json, SendGrid, and Form.

The next line of code isn’t necessary if you’re using plain JavaScript. However, I’m a big fan of TypeScript, so I created a type for handling my errors.

The next section is where all the business logic happens. It’s incredibly straightforward and includes all the lines of code I need to get this working and submit my contact form.

That’s really it! What took me a few hours to figure out in Next.js took me less than an hour of reading the Remix.js documentation to implement. The last thing I changed was removing the action call from the form element that was used in the Next.js code.

This was replaced by the Form component I created in Remix.js.

The end result of this coding challenge was really surprising. I can now see why developers enjoy the experience of writing code in Remix.js.